Ah, git blame
! The detective of Git-land, diligently pointing its finger at the author of each line of code. But sometimes, the blame game can get a little… noisy.
What if you're trying to understand the evolution of a critical piece of logic, but all you see are commits related to code formatting or a massive refactoring that touched almost every file? These commits, while important in their own right, can sometimes obscure the more granular history you're trying to uncover.
Thankfully, there are ways to tell git blame
to politely ignore certain commits, allowing you to focus on the changes that truly matter. This is what this article is all about.
Today, we will explore the situations in which it makes sense to exclude commits and examine our options.
But first, here's a brief overview of the purpose of git blame
.
About the "Git Blame" Command
At its core, git blame
is a Git command that displays the author and revision information for each line of a file.
The primary purpose of this command is to understand the history of a specific piece of code. It helps you answer questions like:
- Who wrote this line of code?
- When was this line last changed?
- Why was this change made (by examining the commit message)?
It works by traversing the commit history of a file; starting from the current version of the file, it looks back through the commits that have modified it, line by line. For each line, it identifies the commit that introduced or last changed that particular line.
Git cleverly tracks line movements across commits. So, if a line of code is moved to a different location within the same file or even to another file (in some cases), git blame
can often still trace its original author and commit.
This makes it a powerful tool for understanding the evolution of your codebase — way more reliable than your colleague John!
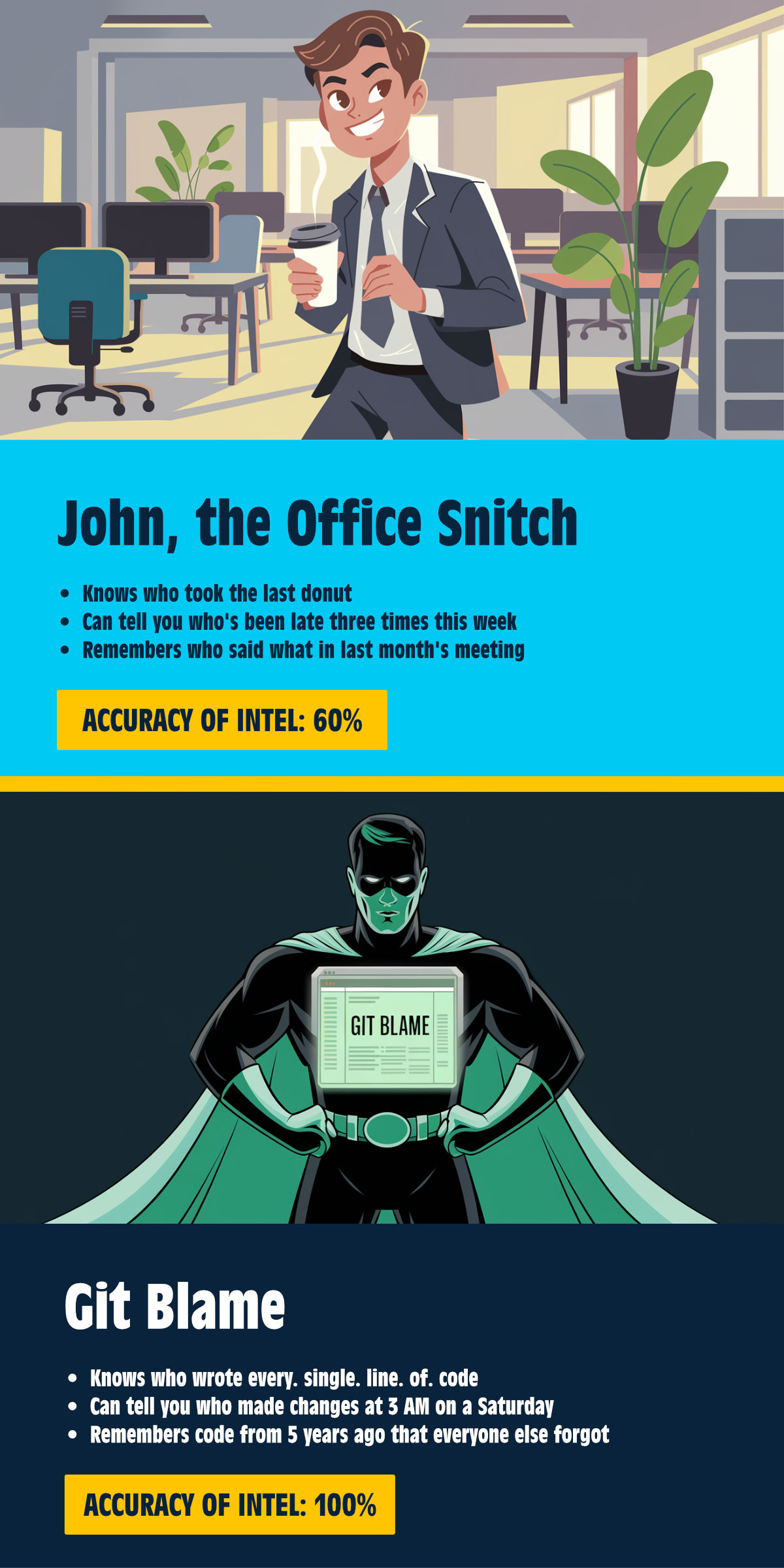
Jokes aside, for every line in the specified file, git blame
shows:
- The commit hash of the last commit that modified that line.
- The author of that commit.
- The date and time of that commit.
- The actual line of code.
This information is essential for debugging, understanding legacy code, and collaborating effectively within a team.
Why Exclude Commits From Git Blame?
While git blame
is incredibly useful, there are scenarios where certain types of commits can clutter the output and make it harder to find the information you're really looking for.
Imagine the following situations:
- Massive Code Formatting: Your team decides to adopt a stricter code style and runs an automated formatter across the entire codebase. This results in a commit that touches almost every line of every file, but doesn't change any functional logic.
Runninggit blame
after this commit might show the formatter as the author of large sections of the code, obscuring the original authors and the actual evolution of the logic. - Large-Scale Refactoring: A significant refactoring effort might involve moving code around, renaming variables, or restructuring directories without fundamentally changing the behavior of the code. These commits can also dominate the
git blame
history, making it difficult to see the more fine-grained changes. - Automated Script Changes: Commits generated by automated scripts, such as dependency updates or generated code, might not provide much useful context when you're trying to understand the human-written parts of the code.
- Cherry-Picks and Merges: Sometimes, cherry-picking or merging commits can result in changes that don't accurately reflect the original authorship in the target branch's history. Excluding the merge commit itself might provide a cleaner view of the individual contributions.
This is where the ability to exclude commits comes in handy. So let's have a look at how it works in practice.
How to Exclude Commits from Git Blame
Git provides a couple of powerful ways to exclude specific commits from the git blame
output:
- Option 1: Creating a
.git-blame-ignore-revs
File - Option 2: Command-Line Options for
git blame
Let's explore both methods.
Option 1: Creating a .git-blame-ignore-revs File
The .git-blame-ignore-revs
file is a configuration file that allows you to specify a list of commit hashes (or SHAs — Secure Hash Algorithm) that git blame
should ignore.
💡 Please note that .git-blame-ignore-revs
is merely a convention; Git does not automatically use this file in the same manner as it does with the .gitignore
file.
However, we recommend using this file name, as popular code hosting platforms like GitHub and GitLab look for and support this file.
1. Creating and Using the File
To use this feature, you first need to create a file named .git-blame-ignore-revs
at the root of your Git repository. This file should contain a list of commit hashes/SHAs, with one hash per line.
For example, your .git-blame-ignore-revs
file might look like this:
# Removed semi-colons from the entire codebase
a1b2c3d4e5f678901234567890abcdef01234567
# Converted all JavaScript to TypeScript
fedcba9876543210fedcba9876543210fedcba98
Each line in this file represents a commit that you want git blame
to skip over when tracing the history of a file. Comments are optional but recommended.
2. Syntax for Listing Commits to Ignore
The syntax for the .git-blame-ignore-revs
file is straightforward: simply list one full commit hash per line. You can obtain these hashes using commands like git log
or by inspecting your Git history in Tower.
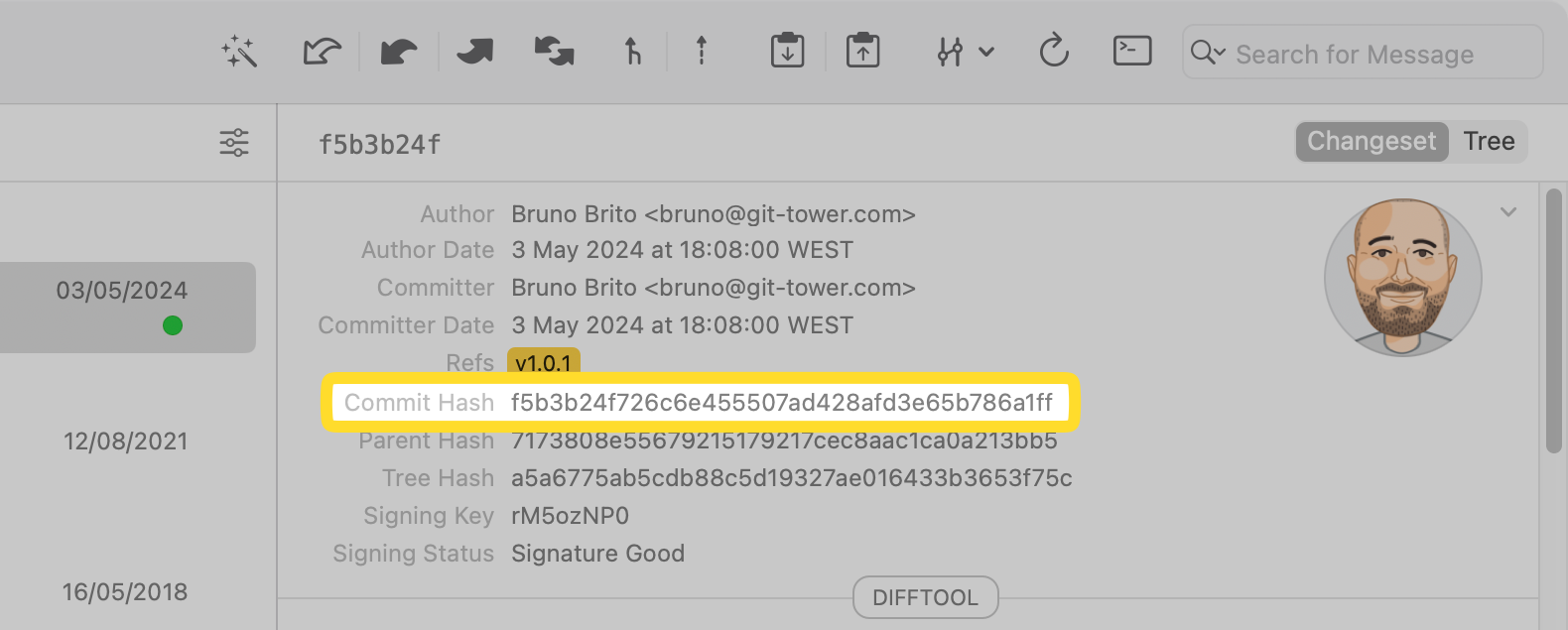
It is important to use the full SHA-1 hash to ensure Git correctly identifies the commits to ignore.
3. Configuring Git to Use the File
Once you've created and populated the .git-blame-ignore-revs
file, you need to tell Git to actually use it. You can do this by setting the blame.ignoreRevsFile
configuration option.
To enable this for your current repository, run the following command in your terminal:
git config blame.ignoreRevsFile .git-blame-ignore-revs
To enable it globally for all your Git repositories, use the --global
flag:
git config --global blame.ignoreRevsFile .git-blame-ignore-revs
With this configuration in place, whenever you run git blame
on a file in your repository (or any repository if you used the --global
option), Git will automatically skip over the commits listed in your .git-blame-ignore-revs
file.
If a line's last modification was in an ignored commit, git blame
will continue to trace the history back to the commit before the ignored one.
Option 2: Command-Line Options for git blame
For more ad-hoc or one-off exclusions, git blame
also provides command-line options to ignore specific commits or an ignore file.
1. The --ignore-rev
Option
The --ignore-rev
option allows you to specify one or more commit hashes/SHAs directly in your git blame
command.
To ignore a single commit, you would use:
git blame --ignore-rev <commit-sha> <file>
To ignore multiple commits, you can use the option multiple times:
git blame --ignore-rev <commit-sha-1> --ignore-rev <commit-sha-2> <file>
This option is useful when you quickly want to exclude a specific commit without modifying the .git-blame-ignore-revs
file.
2. The --ignore-revs-file
Option
If you have a file containing the list of commits to ignore (which doesn't necessarily have to be named .git-blame-ignore-revs
or located at the repository root), you can explicitly tell git blame
to use it with the --ignore-revs-file
option:
git blame --ignore-revs-file <path/to/ignore-file> <file>
This can be helpful if you want to maintain different ignore lists for different purposes or if you prefer to keep the ignore list in a non-standard location.
Best Practices for Excluding Commits
While the ability to exclude commits from git blame
is powerful, it's important to use it judiciously and follow some best practices.
Here are 4 tips you should keep in mind.
1. Maintaining the Ignore List
If you choose to use the .git-blame-ignore-revs
file, it's important to maintain it over time. As your project evolves, you might encounter new formatting or refactoring commits that you want to add to the ignore list.
Consider keeping the ignore list relatively concise and focused on truly disruptive commits. A very long ignore list might indicate a broader issue with your commit history or development practices.
2. Communicating Excluded Commits to the Team
If you are using the repository-specific .git-blame-ignore-revs
file (i.e., you configured blame.ignoreRevsFile
without the --global
flag), it's a good idea to communicate to your team that this file exists and what types of commits are being ignored. This ensures that everyone on the team is aware of the potential differences in their git blame
output.
Since .git-blame-ignore-revs
is typically committed to the repository, it automatically shares the ignore list with everyone who clones the project. However, it's still helpful to mention its existence in your project's documentation or during team meetings.
3. Balancing Exclusion with Accountability
While excluding certain commits can improve the clarity of git blame
output, it's important to maintain accountability. Don't use commit exclusion as a way to hide authorship of problematic code. The primary goal is to improve the signal-to-noise ratio, not to rewrite history or evade responsibility.
Remember that the excluded commits are still part of the Git history and can be examined using other Git commands like git log
. git blame
with exclusions simply provides a filtered view.
4. Integrating Exclusion into Your Workflow
Consider integrating the creation or updating of the .git-blame-ignore-revs
file as part of your team's workflow when performing large-scale formatting or refactoring. For example, the script that performs the formatting could also generate or append to the ignore list.
Using a repository-specific .git-blame-ignore-revs
file and enabling it via git config
within the repository ensures that the ignore rules are automatically applied for everyone working on that project. This provides a consistent and cleaner git blame
experience for the entire team.
Final Words
We've journeyed through the ins and outs of excluding commits from git blame
, from understanding its purpose to mastering the techniques for filtering out noise.
Whether you choose to use the .git-blame-ignore-revs
file for persistent exclusions or the command-line options for more targeted filtering, you now have the tools to make your code history investigations more focused and efficient. So go ahead and use these techniques wisely!
We hope you found this post helpful. For more Git tips and tricks, don't forget to sign up for our newsletter below and follow Tower on Twitter / X and LinkedIn! ✌️
Join Over 100,000 Developers & Designers
Be the first to know about new content from the Tower blog as well as giveaways and freebies via email.